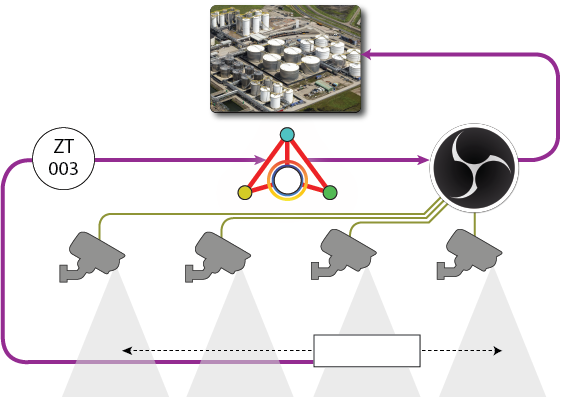
We previously looked at using OBS to create live-data overlays using ARDI. You can also use ARDI to control OBS studio itself, allowing you to switch camera feeds based on live data. You can use this to…
- Track machines or people as they move through your site
- Show the closest camera when alerts or rapid changes in your process are detected
- Switch the camera to the active machines when you have several that move in-and-out of service
- Watch key phases of your work when they occur
How does it work?
We follow a similar setup to our previous article. However, this time we have several different scenes in OBS. These scenes can have different overlays and different camera feeds.
If you’re not using a web overlay, we then write a small Python service application to perform all of the camera-work for us.
If you are already using an ARDI-powered web overlay, you can change cameras using Javascript instead of following this article.
Step 1 – Install & Configure OBS Studio
You’ll need to install OBS Studio and create at least two different scenes.
Depending on which version of OBS Studio you are using, you might need to install the OBS Websocket plugin for OBS Studio.
Step 2 – Create a new Python Script
Create a new, empty Python file, and make sure that ardi.py is in the same directory.
In this case, we have four scenes in OBS, each named after one of the cameras (Camera 1, Camera 2, Camera 3 and Camera 4).
We also have a crane that moves 50m, and we’d like to change the camera to always be viewing the cranes current position. The asset named ‘Crane’ has a single property called ‘Position’, which moves between 0-50m.
from obswebsocket import obsws, requests
import ardi
import traceback
class OBSCameraControl:
def __init__(self):
#Set Defaults
self.host = "localhost"
self.port = 4444
self.password = "myobspassword"
self.ardihost = "localhost"
self.ardisite = "default"
self.asset = "Crane"
self.property = "Position"
pass
def Connect(self):
#Connect to OBS Control Websocket
self.ws = obsws(self.host, self.port, self.password)
self.ws.connect()
#Connect to ARDI live data point
self.srv = ardi.Server(self.ardihost,self.ardisite)
self.srv.Connect()
self.sess = ardi.Session(self.srv)
self.value = self.sess.AddChannel(self.asset,self.property)
self.sess.Callback(self.LiveDataUpdate)
self.sess.Start()
def Disconnect(self):
self.ws.disconnect()
def LiveDataUpdate(self,ld):
if self.value in ld:
#Here is where you put your logic to determine which scene
# you should be viewing
scenename = "Camera 1"
try:
vl = self.value.AsFloat()
if vl > 10:
scenename = "Camera 2"
if vl > 25:
scenename = "Camera 3"
if vl > 40:
scenename = "Camera 4"
except:
traceback.print_exc()
pass
#Set the scene in OBS Studio
try:
print("Switching to Camera: " + scenename)
self.ws.call(requests.SetCurrentScene(scenename))
except:
#If the call fails, OBS or the socket is closed.
try:
self.ws.disconnect()
except:
pass
self.ws = obsws(self.host, self.port, self.password)
try:
self.ws.connect()
except:
print("Unable to connect to OBS")
pass
pass
#Connect to OBS & control with live data
cc = OBSCameraControl()
cc.Connect()
cc.Disconnect()
So this code…
- Connects to the OBS API
- Subscribes to changes in the Crane Position
- When the crane position changes, the position is checked and the appropriate scene is selected
Note that the code above is illustrative and may need some additional tuning and error handling to be production-ready.
Step 3 – Run It
Now you can run the script – your OBS session should now track the moving crane (or do whatever other functions you’ve added) automatically.
Step 4 – Making it Always Run
If you’re setting up an unattended system, you’ll want to run the script as a service.
In Windows you can use NSSM to do this – it can be downloaded, or can is included with your ARDI installation in the drivers/nssm/win64 folder.
On Linux, use your preferred method to launch the script as a daemon.
What more can I do?
With ARDI, you can do almost anything with your live and historical data. If you’d like to find out more, please contact us.